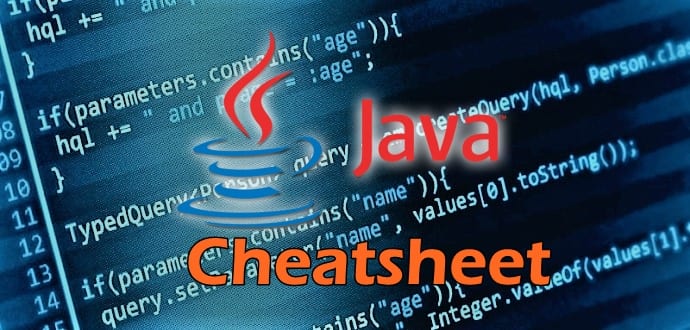
Java Programming Cheatsheet
Java is one of the most popular, most adopted and general purpose programming language used by millions of developers and billions of devices around the world. It is a class-based, object-oriented language and designed to be portable, which means that you can find it on all platforms, operating systems, and devices. It is used to develop all kinds of Android apps, desktop apps, and video games. It is also commonly used as a server-side language for enterprise-level back end development. This programming language has long-term compatibility and developers are comfortable with Java.
Cheat-sheet for Java Programming is given below:
Java Data Types
Data type specifies the size and type of values that can be stored in an identifier. The Java language is rich in its data types. Different data types allow you to select the type appropriate to the needs of the application.
Data types in Java are classified into two types:
Primitive—which include Integer, Character, Boolean, and Floating Point.
Non-primitive—which include Classes, Interfaces, and Arrays.
byte / short / int / long
| 10,-30 |
float / double
| 88.58 |
char
|
‘D’
|
boolean
|
true, false
|
String
|
“Keep Following Techworm”
|
Java Data Conversions
String to Number int i = Integer.parseInt(str); double d = Double.parseDouble(str); |
Any Type to String String s = String.valueOf(value); |
Numeric Conversions int i = (int) |
Java Arithmetic Operators
x + y
|
add
|
x – y
|
subtract
|
x * y
|
multiply
|
x / y
|
divide
|
x % y
|
modulus
|
++x / x++
|
increment
|
–x / x–
|
decrement
|
Java Comparison Operators
x < y
|
Less
|
x <= y
|
Less or eq
|
x > y
|
Greater
|
x >= y
|
Greater or eq
|
x == y
|
Equal
|
x != y
|
Not equal
|
Java Boolean Operators
! x (not)
|
x && y (and)
|
x || y (or)
|
Java Text Formatting
printf style formatting System.out.printf(”Count is %d\n”, count); s = String.format(“Count is %d”, count);MessageFormat style formatting s = MessageFormat.format( ”At {1,time}, {0} Runs Scored.”, 25, new Date());Individual Numbers and Dates s = NumberFormat.getCurrency() .format(x); s = new SimpleDateFormat(“”yyyy/mm/dd””) .format(new Date()); |
Java Hello World
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World"); } } |
Java String Methods
s.length()
|
length of s
|
s.charAt(i)
|
extract ith character
|
s.substring(start, end)
|
substring from start to end-1
|
s.toUpperCase()
|
returns copy of s in ALL CAPS
|
s.toLowerCase()
|
returns copy of s in lowercase
|
s.indexOf(x)
|
index of first occurence of x
|
s.replace(old, new)
|
search and replace
|
s.split(regex)
|
splits string into tokens
|
s.trim()
|
trims surrounding whitespace
|
s.equals(s2)
|
true if s equals s2
|
s.compareTo(s2)
|
0 if equal/+ if s > s2/- if s < s2
|
Java Statements
If Statement
if ( expression ) { statements } else if ( expression ) { statements } else { statements }While Loop while ( expression ) { statements }Do-While Loop do { statements } while ( expression );For Loop for ( int i = 0; i < max; ++i) { statements }For Each Loop for ( var : collection ) { statements }Switch Statement switch ( expression ) { case value: statements break; case value2: statements break; default: statements }Exception Handling try { statements; } catch (ExceptionType e1) { statements; } catch (Exception e2) { catch-all statements; } finally { statements; } |
Applications of Java
Java is being used in:
- Real-time Systems
- Simulation and Modelling
- object oriented Databases
- Artificial Intelligence and Expert Systems
- CIM/CAD/CAM Systems
- Neural Networks and Parallel Programming
- Decision Support Systems
0 comments